When SiriKit was first introduced with iOS 10, an app had to fit neatly into one of the SiriKit domains covered in the chapter entitled “An Introduction to SiriKit” in order to integrate with Siri. In iOS 12, however, SiriKit was extended to allow an app of any type to make key features available for access via Siri voice commands, otherwise known as Siri Shortcuts. This chapter will provide a high level overview of Siri Shortcuts and the steps involved in turning app features into Siri Shortcuts.
An Overview of Siri Shortcuts
A Siri shortcut is essentially a commonly used feature of an app that can be invoked by the user via a chosen phrase. The app for a fast-food restaurant might, for example, allow the user to order a favorite lunch item by simply using the phrase “Order Lunch” from within Siri. Once a shortcut has been configured, iOS learns the usage patterns of the shortcut and will begin to place that shortcut in the Siri Suggestions area on the device at appropriate times of the day. If the user uses the lunch ordering shortcut at lunch times on weekdays, the system will suggest the shortcut at that time of day.
A shortcut can be configured within an app by providing the user with an Add to Siri button at appropriate places in the app. Our hypothetical restaurant app might, for example, include an Add to Siri button on the order confirmation page which, when selected, will allow the user to add that order as a shortcut and provide a phrase to Siri with which to launch the shortcut (and order the same lunch) in future.
An app may also suggest (a concept referred to as donating) a feature or user activity as a possible shortcut candidate which the user can then turn into a shortcut via the iOS Shortcuts app.
As with the SiriKit domains, the key element of a Siri Shortcut is an intent extension. Unlike domain based extensions such as messaging and photo search, which strictly define the parameters and behavior of the intent, Siri shortcut intents are customizable to meet the specific requirements of the app. A restaurant app, for example would include a custom shortcut intent designed to handle information such as the menu items ordered, the method of payment and the pick-up or delivery location. Unlike SiriKit domain extensions, however, the parameters for the intent are stored when the shortcut is created and are not requested from the user by Siri when the shortcut is invoked. A shortcut to order the user’s usual morning coffee, for example, will already be configured with the coffee item and pickup location, both of which will be passed to the intent handler by Siri when the shortcut is triggered.
The intent will also need to be configured with custom responses such as notifying the user through Siri that the shortcut was successfully completed, a particular item in an order is currently unavailable or that the pickup location is currently closed.
The key component of a Siri Shortcut in terms of specifying the parameters and responses is the SiriKit Intent Definition file.
An Introduction to the Intent Definition File
When a SiriKit extension such as a Messaging Extension is added to an iOS project, it makes use of a pre-defined system intent provided by SiriKit (in the case of a Messaging extension, for example, this might involve the INSendMessageIntent). In the case of Siri shortcuts, however, a custom intent is created and configured to fit with the requirements of the app. The key to creating a custom intent lies within the Intent Definition file.
An Intent Definition file can be added to an Xcode project by selecting the Xcode File -> New -> File… menu option and choosing the SiriKit Intent Definition File option from the Resource section of the template selection dialog.
Once created, Xcode provides an editor specifically for adding and configuring custom intents (in fact the editor may also be used to create customized variations of the system intents such as the Messaging, Workout and Payment intents). New intents are added by clicking on the ‘+’ button in the lower left corner of the editor window and making a selection from the menu:
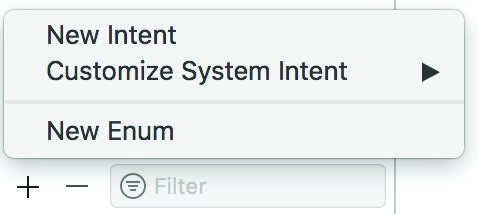
Figure 45-1
Selecting the Customize System Intent option will provide a list of system intents from which to choose while the New Intent option creates an entirely new intent with no pre-existing configuration settings. Once a new custom intent has been created, it appears in the editor as shown in Figure 45-2:
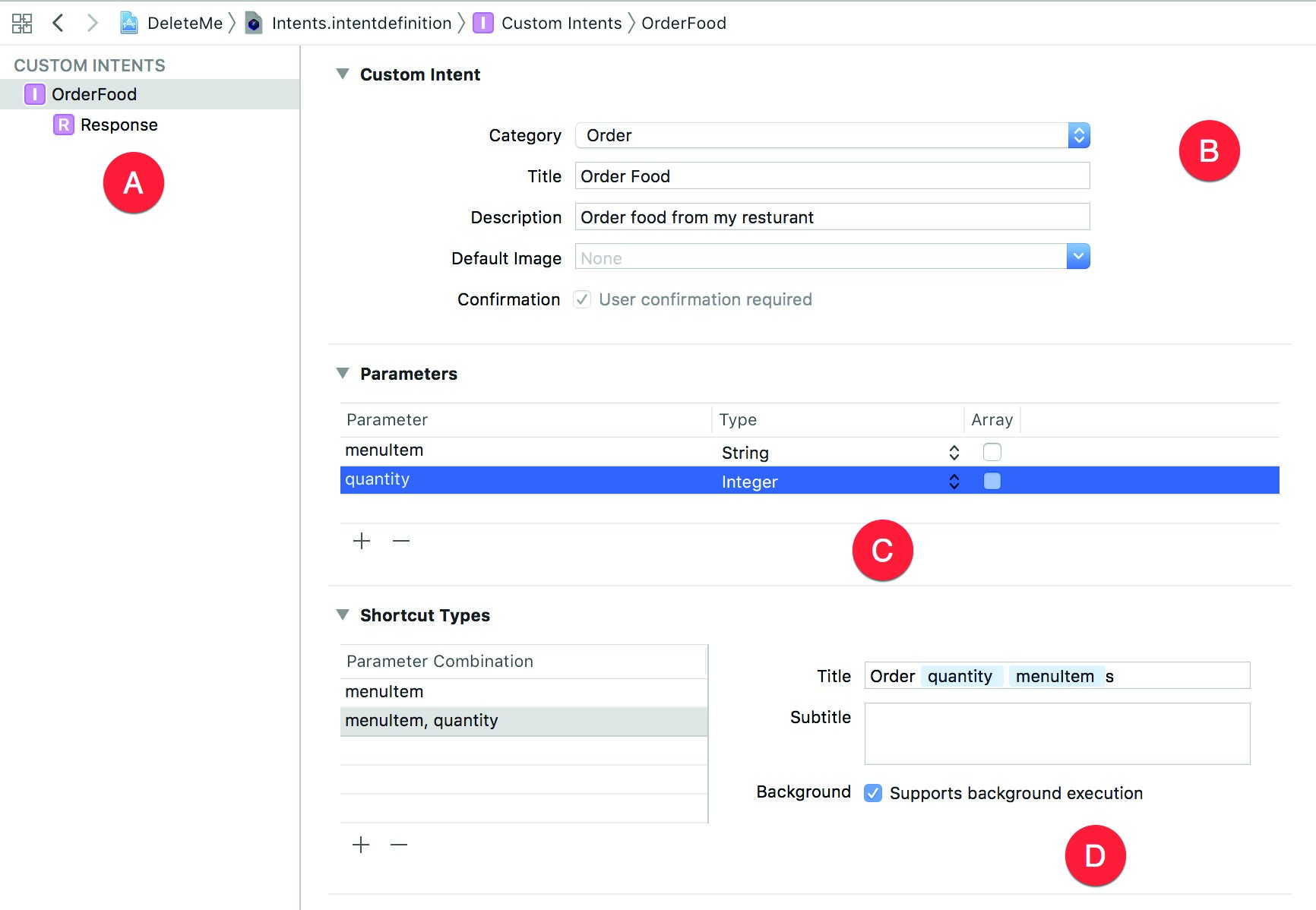
Figure 45-2
The section marked A in the above figure lists the custom intents contained within the file. The Custom Intent section (B) is where the category of the intent is selected from a list of options including categories such as order, buy, do, open, search etc. Since this example is an intent for ordering food, the category is set to Order. This section also includes the title of the intent, a description and an optional image to include with the shortcut. A checkbox is also provided to require Siri to seek confirmation from the user before completing the shortcut. For some intent categories such as buying and ordering this option is mandatory.
The parameters section (C) allows the parameters that will be passed to the intent to be declared. If the custom intent is based on an existing SiriKit system intent, this area will be populated with all of the parameters the intent is already configured to handle and the settings cannot be changed. For a new custom intent, as many parameters as necessary may be declared and configured in terms of name, type and whether or not the parameter is an array.
Finally, the Shortcut Types section (D) allows different variations of the shortcut to be configured. A shortcut type is defined by the combination of parameters used within the shortcut. For each combination of parameters that the shortcut needs to support, settings are configured including the title of the shortcut and whether or not the host app can perform the associated task in the background without presenting a user interface. Each intent can have as many parameter combinations as necessary to provide a flexible user shortcut experience.
The Custom Intents panel (A) also lists a Response entry under the custom intent name. When selected, the editor displays the screen shown in Figure 45-3:
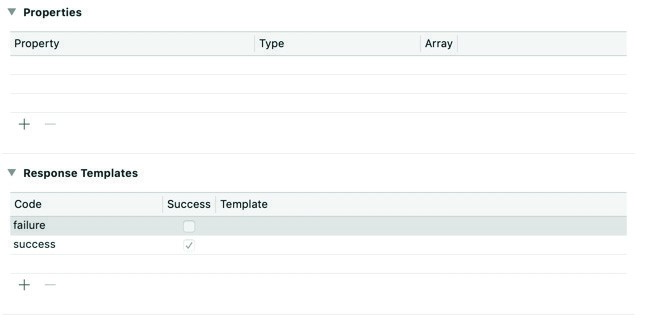
Figure 45-3
Keep in mind that a Siri shortcut involves a two-way interaction between Siri and the user and that to maintain this interaction Siri needs to know how to respond to the user based on the results of the intent execution. This is defined through a range of response templates which define the phrases to be spoken by Siri when the intent returns specific codes. These response settings also allow parameters to be configured for inclusion in the response phrases. A restaurant app might, for example, have a response code named failureStoreClosed which results in Siri responding with the phrase “We are sorry, we cannot complete your cheeseburger order because the store is closed.”, and a success response code with the phrase “Your order of two coffees will be ready for pickup in 20 minutes”.
Once the Intent Definition file has been configured, Xcode will automatically generate a set of class files ready for use within the intent handler code of the extension.
Automatically Generated Classes
The purpose of the Intent Definition file is to allow Xcode to generate a set of classes that will be used when implementing the shortcut extension. Assuming that a custom intent named OrderFood was added to the definition file, the following classes would be automatically generated by Xcode:
- OrderFoodIntent – The intent object that encapsulates all of the parameters declared in the definition file. An instance of this class will be passed by Siri to the handler(), handle() and confirm() methods of the extension intent handler configured with the appropriate parameter values for the current shortcut.
- OrderIntentHandling – Defines the protocol to which the intent handler must conform in order to be able to fully handle food ordering intents.
- OrderIntentResponse – A class encapsulating the response codes, templates and parameters declared for the intent in the Intent Definition file. The intent handler will use this class to return response codes and parameter values to Siri so that the appropriate response can be communicated to the user.
Use of these classes within the intent handler will be covered in the next chapter entitled “A SwiftUI Siri Shortcut Tutorial”.
Donating Shortcuts
An app typically donates a shortcut when a common action is performed by the user. This donation does not automatically turn the activity into a shortcut, but includes it as a suggested shortcut within the iOS Shortcuts app. A donation is made by calling the donate() method of an INInteraction instance which has been initialized with a shortcut intent object. For example:
let intent = OrderFoodIntent() intent.menuItem = "Cheeseburger" intent.quantity = 1 intent.suggestedInvocationPhrase = "Order Lunch" let interaction = INInteraction(intent: intent, response: nil) interaction.donate { (error) in if error != nil { // Handle donation failure } }
The Add to Siri Button
The Add to Siri button allows a shortcut to be added to Siri directly from within an app. This involves writing code to create an INUIAddVoiceShortcutButton instance, initializing it with a shortcut intent object with shortcut parameters configured and then adding it to a user interface view. A target method is then added to the button to be called when the button is clicked.
As of iOS 14, the Add to Siri button has not been integrated directly into SwiftUI, requiring the integration of UIKit into the SwiftUI project.
Summary
Siri shortcuts allow commonly performed tasks within apps to be invoked using Siri via a user provided phrase. When added, a shortcut contains all of the parameter values needed to complete the task within the app together with templates defining how Siri should respond based on the status reported by the intent handler. A shortcut is implemented by creating a custom intent extension and configuring an Intent Definition file containing all of the information regarding the intent, parameters and responses. From this information, Xcode generates all of the class files necessary to implement the shortcut. Shortcuts can be added to Siri either via an Add to Siri button within the host app, or by the app donating suggested shortcuts. A list of donated shortcuts can be found in the iOS Shortcuts app.