In the previous chapter, we explored how to integrate in-app purchasing into an Android project and also looked at some code samples that can be used when working on your own projects. This chapter will put this theory into practice by creating an example project demonstrating how to add a consumable in-app product to an Android app using Jetpack Compose. The tutorial will also show how in-app products are added and managed within the Google Play Console and explain how to enable test payments so that purchases can be made during testing without having to spend real money.
About the In-App Purchasing Example Project
The simple concept behind this project is an app in which an in-app product must be purchased before a button can be clicked. This in-app product is consumed each time the button is clicked, requiring the user to re-purchase the product each time they want to be able to click the button. On initialization, the app will connect to the app store, obtain details of the product, and display the product name. Once the app has established that the product is available, a purchase button will be enabled which, when clicked, will step through the purchase process. On completion of the purchase, a second button will be enabled so that the user can click on it and consume the purchase.
Creating the InAppPurchase Project
The first step in this exercise is to create a new project. Begin by launching Android Studio and selecting the New Project option from the welcome screen. In the new project dialog, choose the Empty Compose Activity template before clicking on the Next button.
Enter InAppPurchase into the Name field and specify a package name that will uniquely identify your app within the Google Play ecosystem (for example com.<your company>.InAppPurchase). Before clicking on the Finish button, change the Minimum API level setting to API 26: Android 8.0 (Oreo).
Within the MainActivity.kt file, delete the Greeting function and add a new empty composable named MainScreen:
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |
@Composable
fun MainScreen() {
}
Code language: Kotlin (kotlin)
Next, edit the onCreateActivity() method function to call MainScreen instead of Greeting. Since this project will be using features that are not supported by the Preview panel, also delete the DefaultPreview composable from the file. To test the project we will be running it on a device or emulator session.
Adding Libraries to the Project
Before we start writing code, some libraries need to be added to the project build configuration, including the standard Android billing client libraries. Later in the project, we will also need to use the ImmutableList class which is part of Google’s Guava Core Java libraries. Add these libraries now by modifying the Gradle Scripts -> build.gradle (Module: InAppPurchase.app) file with the following changes:
.
.
dependencies {
.
.
implementation 'com.android.billingclient:billing:6.0.1'
implementation 'com.android.billingclient:billing-ktx:6.0.1'
implementation 'com.google.guava:guava:24.1-jre'
implementation 'com.google.guava:guava:27.0.1-android'
.
.
Code language: Gradle (gradle)
Click on the Sync Now link at the top of the editor panel to commit these changes.
Adding the App to the Google Play Store
Using the steps outlined in the chapter entitled Creating, Testing, and Uploading an Android App Bundle, sign into the Play Console, create a new app, and set up a new internal testing track including the email addresses of designated testers. Return to Android Studio and generate a signed release app bundle for the project. Once the bundle file has been generated, upload it to the internal testing track and roll it out for testing.
Now that the app has a presence in the Google Play Store, we are ready to create an in-app product for the project.
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |
Creating an In-App Product
With the app selected in the Play Console, scroll down the list of options in the left-hand panel until the Monetize section comes into view. Within this section, select the In-app products option listed under Products as shown in Figure 1-1:
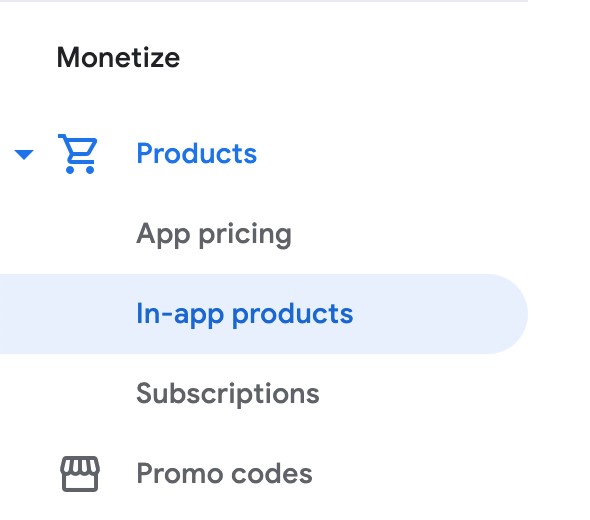
Figure 1-1
On the In-app products page, click on the Create product button:

Figure 1-2
On the new product screen, enter the following information before saving the new product:
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |
- Product ID: one_button_click
- Name: A Button Click
- Description: This is a test in-app product that allows a button to be clicked once.
- Default price: Set to the lowest possible price in your preferred currency.
Enabling License Testers
When testing in-app billing it is useful to be able to make test purchases without spending any money. This can be achieved by enabling license testing for the internal track testers. License testers can use a test payment card when making purchases so that they are not charged.
Within the Play Console, return to the main home screen and select the Setup -> License testing option:
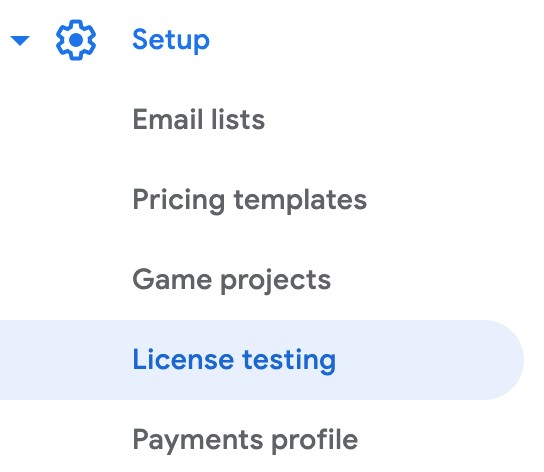
Figure 1-3
Within the license testing screen, add the testers that were added for the internal testing track, change the License response setting to RESPOND_NORMALLY, and save the changes:
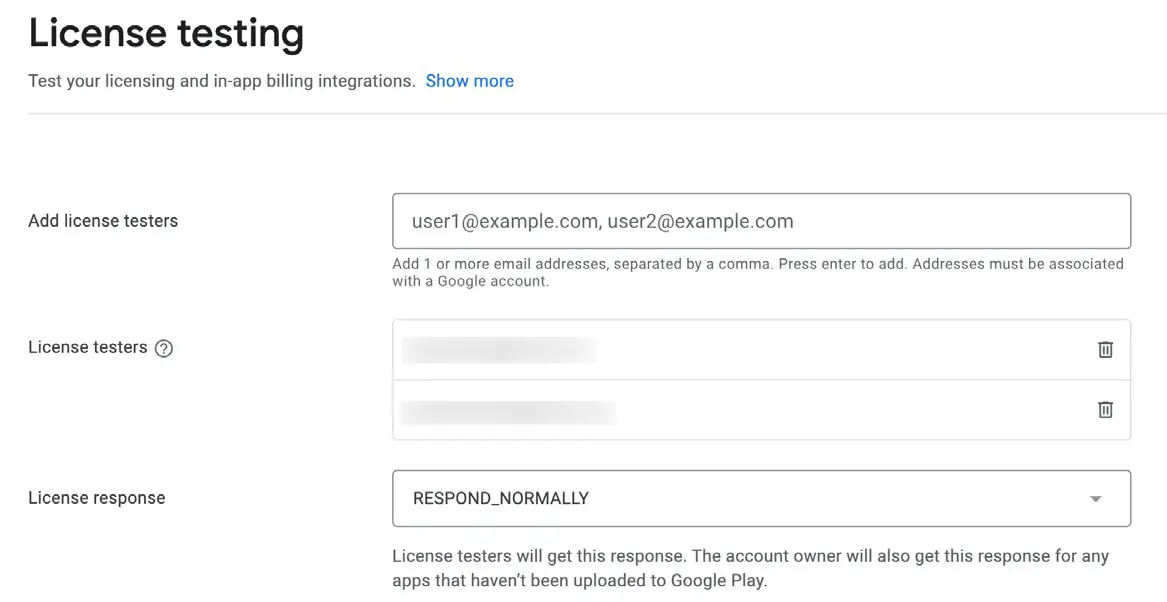
Figure 1-4
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |
Now that both the app and the in-app product have been set up in the Play Console, we can start adding code to the project.
Creating a Purchase Helper Class
To establish a clean separation between the user interface and billing code, we will create a new helper class that will handle all of the purchasing tasks and use StateFlow instances to update the user interface with status changes. While it may be tempting to create this helper class as a view model, doing so will result in unstable code. The problem is that the billing client will need a reference to the main activity to process purchase transactions. This means that we will need to pass this reference to our helper class when an instance is created. As we know from previous chapters, activities are subject to being destroyed and recreated during the lifecycle of an app. Since view models are, by definition, designed to survive the destruction and recreation of activities we run the risk within our billing code of relying on a reference to an activity that no longer exists. To avoid this problem we will declare our purchase helper as a standard Kotlin data class that will be destroyed and recreated along with the activity.
Within the Project tool window, right-click on the com.<your company>.InAppPurchase entry, select the New -> Kotlin Class/File menu option and create a new class named PurchaseHelper. With the new class file created, edit it so that it reads as follows:
.
.
import android.app.Activity
import android.util.Log
import com.android.billingclient.api.*
import com.google.common.collect.ImmutableList
import kotlinx.coroutines.CoroutineScope
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.flow.MutableStateFlow
import kotlinx.coroutines.flow.asStateFlow
import kotlinx.coroutines.launch
data class PurchaseHelper(val activity: Activity) {
}
Code language: Kotlin (kotlin)
These changes import a set of libraries that will be needed later in the chapter and configure the class to expect an Activity initialization parameter. Next, add variable declarations to store values related to the billing process together with the id of the product created in the Google Play Console:
.
.
data class PurchaseHelper(val activity: Activity) {
private val coroutineScope = CoroutineScope(Dispatchers.IO)
private lateinit var billingClient: BillingClient
private lateinit var productDetails: ProductDetails
private lateinit var purchase: Purchase
private val demoProductId = "one_button_click"
.
.
Code language: Kotlin (kotlin)
Adding the StateFlow Streams
Communication between the purchase process and the user interface will be performed using StateFlow streams. Specifically, the user interface will use these to display status information on Text components and to ensure that Buttons are appropriately enabled and disabled. Using the techniques outlined in the chapter titled Kotlin Flow with Jetpack Compose, add the following StateFlow declarations to the PurchaseHelper class:
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |
data class PurchaseHelper(val activity: Activity) {
.
.
private val _productName = MutableStateFlow("Searching...")
val productName = _productName.asStateFlow()
private val _buyEnabled = MutableStateFlow(false)
val buyEnabled = _buyEnabled.asStateFlow()
private val _consumeEnabled = MutableStateFlow(false)
val consumeEnabled = _consumeEnabled.asStateFlow()
private val _statusText = MutableStateFlow("Initializing...")
val statusText = _statusText.asStateFlow()
}
Code language: Kotlin (kotlin)
Initializing the Billing Client
Next, the PurchaseHelper class needs a method that can be called from the MainActivity to initialize the billing client. Remaining within the PurchaseHelper.kt file, add this new method as follows:
fun billingSetup() {
billingClient = BillingClient.newBuilder(activity)
.setListener(purchasesUpdatedListener)
.enablePendingPurchases()
.build()
billingClient.startConnection(object : BillingClientStateListener {
override fun onBillingSetupFinished(
billingResult: BillingResult
) {
if (billingResult.responseCode ==
BillingClient.BillingResponseCode.OK
) {
_statusText.value = "Billing Client Connected"
queryProduct(demoProductId)
} else {
_statusText.value = "Billing Client Connection Failure"
}
}
override fun onBillingServiceDisconnected() {
_statusText.value = "Billing Client Connection Lost"
}
})
}
Code language: Kotlin (kotlin)
When this method is called, it will create a new billing client instance and attempt to connect to the Google Play Billing Library. The onBillingSetupFinished() listener will be called when the connection attempt completes and update the statusText state flow indicating the success or otherwise of the connection attempt. Finally, we have also implemented the onBillingServiceDisconnected() callback which will be called if the Google Play Billing Library connection is lost.
If the connection is successful a method named queryProduct() is called. Both this method and the purchasesUpdatedListener assigned to the billing client now need to be added.
Querying the Product
To make sure the product is available for purchase, we need to create a QueryProductDetailsParams instance configured with the product ID that was specified in the Play Console, and pass it to the queryProductDetailsAsync() method of the billing client. This will require that we also add the onProductDetailsResponse() callback method where we will check that the product exists, extract the product name, and assign it to the statusText state. Now that we have obtained the product details, we can also safely enable the purchase button via the buyEnabled flow. Within the PurchaseHelper.kt file, add the queryProduct() method so that it reads as follows:
fun queryProduct(productId: String) {
val queryProductDetailsParams = QueryProductDetailsParams.newBuilder()
.setProductList(
ImmutableList.of(
QueryProductDetailsParams.Product.newBuilder()
.setProductId(productId)
.setProductType(
BillingClient.ProductType.INAPP
)
.build()
)
)
.build()
billingClient.queryProductDetailsAsync(
queryProductDetailsParams
) { billingResult, productDetailsList ->
if (productDetailsList.isNotEmpty()) {
productDetails = productDetailsList[0]
_productName.value = "Product: " + productDetails.name
} else {
_statusText.value = "No Matching Products Found"
_buyEnabled.value = false
}
}
}
Code language: Kotlin (kotlin)
Much of the code used here should be familiar from the previous chapter. The listener code checks that at least one product was found that matches the query criteria. The ProductDetails object is then extracted from the first matching product, stored in the productDetails variable, and the product name property assigned to the productName state flow.
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |
Handling Purchase Updates
The results of the purchase process will be reported to the app via the PurchaseUpdatedListener that was assigned to the billing client during the initialization phase. Add this handler now as follows:
private val purchasesUpdatedListener =
PurchasesUpdatedListener { billingResult, purchases ->
if (billingResult.responseCode ==
BillingClient.BillingResponseCode.OK
&& purchases != null
) {
for (purchase in purchases) {
completePurchase(purchase)
}
} else if (billingResult.responseCode ==
BillingClient.BillingResponseCode.USER_CANCELED
) {
_statusText.value = "Purchase Canceled"
} else {
_statusText.value = "Purchase Error"
}
}
Code language: Kotlin (kotlin)
The handler will update the status text if the user cancels the purchase or another error occurs. A successful purchase, however, results in a call to a method named completePurchase() which is passed the current Purchase object. Add this method as outlined below:
private fun completePurchase(item: Purchase) {
purchase = item
if (purchase.purchaseState == Purchase.PurchaseState.PURCHASED) {
_buyEnabled.value = false
_consumeEnabled.value = true
_statusText.value = "Purchase Completed"
}
}
Code language: Kotlin (kotlin)
This method stores the purchase before verifying that the product has indeed been purchased and that payment is not still pending. The consume button is enabled, the purchase button disabled, and the user is notified that the purchase was successful.
Launching the Purchase Flow
We now need to add the following method which will be called from the purchase button in the user interface to start the purchase process:
fun makePurchase() {
val billingFlowParams = BillingFlowParams.newBuilder()
.setProductDetailsParamsList(
ImmutableList.of(
BillingFlowParams.ProductDetailsParams.newBuilder()
.setProductDetails(productDetails)
.build()
)
)
.build()
billingClient.launchBillingFlow(activity, billingFlowParams)
}
Code language: Kotlin (kotlin)
Consuming the Product
With the user now able to click on the “consume” button, the next step is to make sure the product is consumed so that only one click can be performed before another button click is purchased. This requires that we now write the consumePurchase() method:
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |
fun consumePurchase() {
val consumeParams = ConsumeParams.newBuilder()
.setPurchaseToken(purchase.purchaseToken)
.build()
coroutineScope.launch {
val result = billingClient.consumePurchase(consumeParams)
if (result.billingResult.responseCode ==
BillingClient.BillingResponseCode.OK) {
_statusText.value = "Purchase Consumed"
_buyEnabled.value = true
_consumeEnabled.value = false
}
}
}
Code language: Kotlin (kotlin)
This method creates a ConsumeParams instance and configures it with the purchase token for the current purchase (obtained from the Purchase object previously saved in the completePurchase() method). This is passed to the consumePurchase() method which is launched within a coroutine using the IO dispatcher. If the product is successfully consumed, the consume button is disabled and the status text updated.
Restoring a Previous Purchase
With the code added so far, we can purchase a product and consume it within a single session. If we were to make a purchase and then exit the app before consuming it the purchase would currently be lost when the app restarts. We can solve this problem by configuring a QueryPurchasesParams instance to search for the unconsumed In-App product and passing it to the queryPurchasesAsync() method of the billing client together with a reference to a listener that will be called with the results. Add a new method and the listener to the MainActivity.kt file as follows:
private fun reloadPurchase() {
val queryPurchasesParams = QueryPurchasesParams.newBuilder()
.setProductType(BillingClient.ProductType.INAPP)
.build()
billingClient.queryPurchasesAsync(
queryPurchasesParams,
purchasesListener
)
}
private val purchasesListener =
PurchasesResponseListener { billingResult, purchases ->
if (purchases.isNotEmpty()) {
purchase = purchases.first()
_buyEnabled.value = false
_consumeEnabled.value = true
_statusText.value = "Previous Purchase Found"
} else {
_buyEnabled.value = true
_consumeEnabled.value = false
}
}
Code language: PHP (php)
If the list of purchases passed to the listener is not empty, the first purchase in the list is assigned to the purchase variable, and the consume button enabled (in a more complete implementation code should be added to check this is the correct product by comparing the product id and to handle the return of multiple purchases). If no purchases are found, the consume button is disabled until another purchase is made. All that remains is to call our new reloadPurchase() method during the billing setup process as follows:
fun billingSetup() {
.
.
if (billingResult.responseCode ==
BillingClient.BillingResponseCode.OK
) {
_statusText.value = "Billing Client Connected"
queryProduct(demoProductId)
reloadPurchase()
} else {
_statusText.value = "Billing Client Connection Failure"
}
}
.
.
}
Code language: Kotlin (kotlin)
Completing the MainActivity
Now that the helper class is completed, changes need to be made to the MainActivity.kt file. The first step is to modify the onCreate() function to create an instance of our PurchaseHelper class and pass it to the MainScreen composable:
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
InAppPurchaseTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
val purchaseHelper = PurchaseHelper(this)
purchaseHelper.billingSetup()
MainScreen(purchaseHelper)
}
}
}
}
Code language: Kotlin (kotlin)
Remaining in the MainActivity.kt file, modify the MainScreen function as follows to accept the purchase handler instance and to collect from the state flow instances:
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |
.
.
import androidx.compose.runtime.*
.
.
@Composable
fun MainScreen(purchaseHelper: PurchaseHelper) {
val buyEnabled by purchaseHelper.buyEnabled.collectAsState(false)
val consumeEnabled by purchaseHelper.consumeEnabled.collectAsState(false)
val productName by purchaseHelper.productName.collectAsState("")
val statusText by purchaseHelper.statusText.collectAsState("")
}
Code language: Kotlin (kotlin)
The final task before testing the app is to call the composables that make up the user interface. This will consist of a Column containing two Text components and an embedded Row containing two Buttons configured to call the makePurchase() and consumePurchase() methods of the purchase handler. The content displayed by the Text composables and the status of the buttons will be controlled by the state flow values. Make the following changes to complete the MainScreen composable:
.
.
import androidx.compose.foundation.layout.*
import androidx.compose.ui.Alignment
import androidx.compose.material.Button
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
.
.
@Composable
fun MainScreen(purchaseHelper: PurchaseHelper) {
.
.
Column(
Modifier.padding(20.dp),
horizontalAlignment = Alignment.CenterHorizontally,
verticalArrangement = Arrangement.Center
) {
Text(
productName,
Modifier.padding(20.dp),
fontSize = 30.sp)
Text(statusText)
Row(Modifier.padding(20.dp)) {
Button(
onClick = { purchaseHelper.makePurchase() },
Modifier.padding(20.dp),
enabled = buyEnabled
) {
Text("Purchase")
}
Button(
onClick = { purchaseHelper.consumePurchase() },
Modifier.padding(20.dp),
enabled = consumeEnabled
) {
Text("Consume")
}
}
}
}
Code language: Kotlin (kotlin)
Testing the App
Before we can test the app we need to upload this latest version to the Play Console. As we already have version 1 uploaded, we first need to increase the version number in the build.gradle (Module: InAppPurchase.app) file:
.
.
defaultConfig {
applicationId "com.ebookfrenzy.inapppurchase"
minSdk 26
targetSdk 32
versionCode 2
versionName "2.0"
.
.
Code language: Gradle (gradle)
Sync the build configuration, then follow the steps in the Creating, Testing, and Uploading an Android App Bundle chapter to generate a new app bundle, upload it to the internal test track and roll it out to the testers. Using the testing track link, install the app on a device or emulator on which one of the test accounts is signed in.
After the app starts the user interface should appear as shown in Figure 1-5 below with the billing client connected, the product name displayed, and the Purchase button enabled:
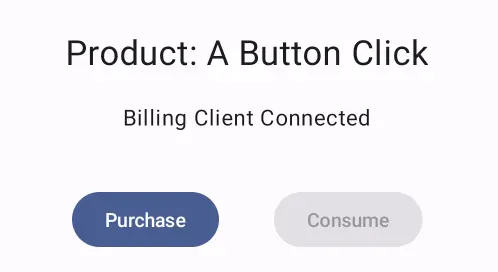
Figure 1-5
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |
Clicking the Purchase button will begin the purchase flow as shown in Figure 1-6:
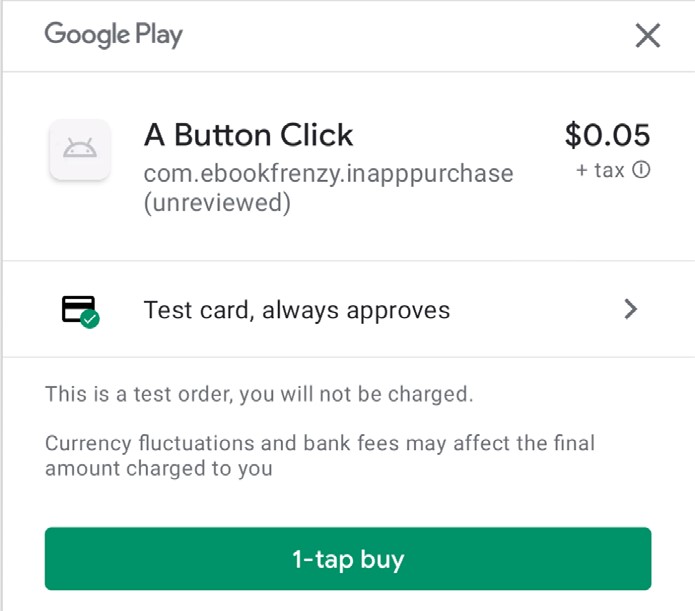
Figure 1-6
Tap the buy button to complete the purchase using the test card and wait for the Consume button to be enabled.
Tap the Consume button and wait for the “Purchase Consumed” status message to appear. With the product consumed, it should now be possible to purchase it again. Make another purchase, then terminate and restart the app. The app should locate the previous unconsumed purchase and enable the consume button.
Troubleshooting
For additional information about failures, a useful trick is to access the debug message from BillingResult instances, for example:
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |
.
.
} else if (billingResult.responseCode ==
BillingClient.BillingResponseCode.USER_CANCELED
) {
_statusText.value = "Purchase Canceled"
} else {
_statusText.value = "Purchase Error"
Log.i("InAppPurchase", billingResult.getDebugMessage())
}
Code language: Kotlin (kotlin)
After adding the debug code, make sure the device is attached to Android Studio, either via a USB cable or WiFi, and select it from within the Logcat panel. Enter InAppPurchaseTag into the Logcat search bar and check the diagnostic output, adding additional Log calls in the code if necessary.
Note that as long as you leave the app version number unchanged in the module-level build.gradle file, you should now be able to run modified versions of the app directly on the device or emulator without having to re-bundle and upload it to the console.
If the test payment card is not listed, make sure the user account on the device has been added to the license testers list. If the app is running on a physical device, try running it on an emulator. If all else fails, you can enter a valid payment method to make test purchases, and then refund yourself using the Order management screen accessible from the Play Console home page.
Summary
In this chapter, we created a project that demonstrated how to add an in-app product to an Android app. This included the creation of the product within the Google Play Console and the writing of code to initialize and connect to the billing client, querying of available products, and, finally, the purchase and consumption of the product. We also explained how to add license testers using the Play Console so that purchases can be made during testing without spending money.