Extensions are a feature originally introduced as part of the iOS 8 release designed to allow certain capabilities of an app to be made available for use within other apps. For example, the developer of a photo editing app might have devised some unique image filtering capabilities and decided that those features would be particularly useful to users of the iOS Photos app. To achieve this, the developer would implement these features in a Photo Editing extension which would then appear as an option to users when editing an image within the Photos app.
Extensions fall into various categories, and several rules and guidelines must be followed in the implementation process. While subsequent chapters will cover the creation of extensions of various types in detail, this chapter is intended to serve as a general overview and introduction to the subject of extensions in iOS.
iOS Extensions – An Overview
The sole purpose of an extension is to make a specific feature of an existing app available for access within other apps. Extensions are separate executable binaries that run independently of the corresponding app. Although extensions are individual binaries, they must be supplied and installed as part of an app bundle. The app with which an extension is bundled is called the containing app. Except for Message App extensions, the containing app must provide useful functionality. An empty app must not be provided solely to deliver an extension to the user.
Once an extension has been installed, it will be accessible from other apps through various techniques depending on the extension type. The app from which an extension is launched and used is referred to as a host app.
For example, an app that translates text to a foreign language might include an extension that can be used to translate the text displayed by a host app. In such a scenario, the user would access the extension via the Share button in the host app’s user interface, and the extension would display a view controller displaying the translated text. On dismissing the extension, the user is returned to the host app.
Extension Types
iOS supports several different extension types dictated by extension points. An extension point is an area of the iOS operating system that has been opened up to allow extensions to be implemented. When developing an extension, it is important to select the extension point most appropriate to the extension’s features. The extension types supported by iOS are constantly evolving, though the key types can be summarized as follows:
Share Extension
Share extensions provide a quick access mechanism for sharing content such as images, videos, text, and websites within a host app with social network sites or content-sharing services. It is important to understand that Apple does not expect developers to write Share extensions designed to post content to destinations such as Facebook or Twitter (such sharing options are already built into iOS) but rather as a mechanism to make sharing easier for developers hosting their own sharing and social sites. Share extensions appear within the activity view controller panel when the user taps the Share button from within a host app.
Share extensions can use the SLComposeServiceViewController class to implement the interface for posting content. This class displays a view containing a preview of the information to be posted and provides the ability to modify the content before posting it. In addition, a custom user interface can be designed using Interface Builder for more complex requirements.
The actual mechanics of posting the content will depend on how the target platform works.
Action Extension
The Action extension point enables extensions to be created that fall into the Action category. Action extensions allow the content within a host app to be transformed or viewed differently. As with Share extensions, Action extensions are accessed from the activity view controller via the Share button. Figure 75-1, for example, shows an example Action extension named “Change It Up” in the activity view controller of the iOS Notes app.
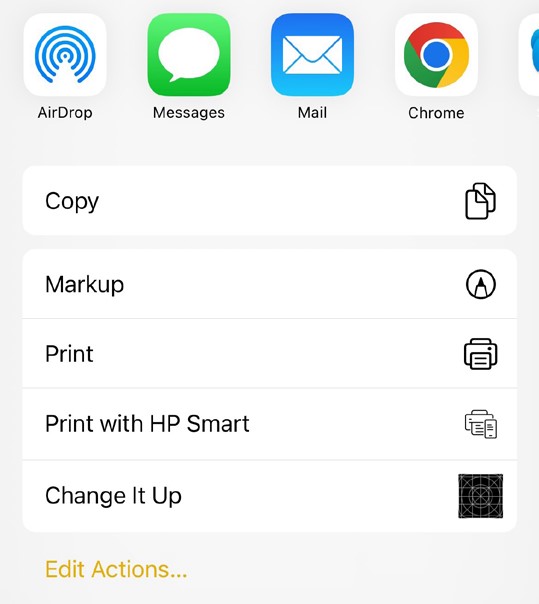
Action extensions are context-sensitive in that they only appear as an option when the type of content in the host app matches one of the content types for which the extension has declared support. For example, an Action extension that works with images will not appear in the activity view controller panel for a host app displaying text-based content.
Action extensions are covered in detail in the chapters entitled Creating an iOS 17 Action Extension and Receiving Data from an iOS 17 Action Extension.
Photo Editing Extension
The Photo Editing extension allows an app’s photo editing capabilities to be accessed from within the built-in iOS Photos app. Photo Editing extensions are displayed when the user selects an image in the Photos app, chooses the edit option, and taps on the button in the top left-hand corner of the Photo editing screen. For example, Figure 75-2 shows the Photos app displaying two Photo Editing extension options:
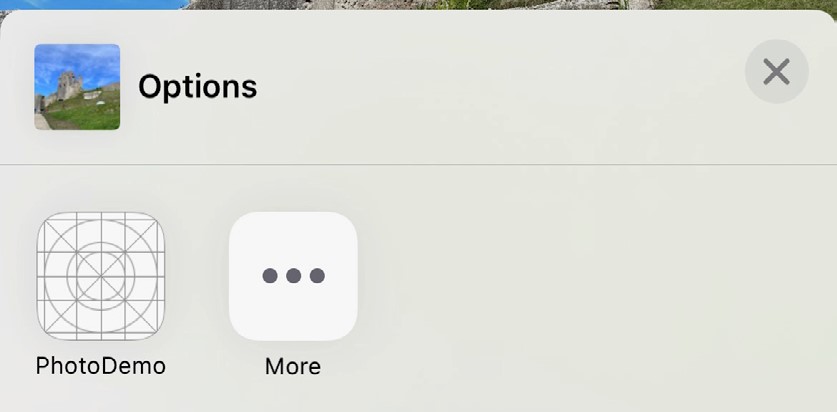
Photo Editing Extensions are covered in detail in the chapter entitled Creating an iOS 17 Photo Editing Extension.
Document Provider Extension
The Document Provider extension allows a containing app to act as a document repository for other apps running on the device. Depending on the level of support implemented in the extension, host apps can import, export, open, and move documents to and from the storage repository the containing app provides. In most cases, the storage repository represented by the containing app will be a third-party cloud storage service providing an alternative to Apple’s iCloud service.
A Document Provider extension consists of a Document Picker View Controller extension and an optional File Provider extension. The Document Picker View Controller extension provides a user interface, allowing users to browse and select the documents available for the Document Provider extension.
The optional File Provider extension provides the host app with access to the documents outside the app’s sandbox and is necessary if the extension is to support move and open operations on the documents stored via the containing app.
Custom Keyboard Extension
As the name suggests, the Custom Keyboard Extension allows creating and installing custom keyboards onto iOS devices. Keyboards developed using the Custom Keyboard extension point are available to be used by all apps on the device and, once installed, are selected from within the keyboard settings section of the Settings app on the device.
Audio Unit Extension
Audio Unit Extensions allow sound effects, virtual instruments, and other sound-based capabilities to be available to other audio-oriented host apps such as GarageBand.
Shared Links Extension
The Shared Links Extension provides a mechanism for an iOS app to store URL links in the Safari browser shared links list.
Content Blocking Extension
Content Blocking allows extensions to be added to the Safari browser to block certain types of content from appearing when users browse the web. This feature is typically used to create ad-blocking solutions.
Sticker Pack Extension
An extension to the built-in iOS Messages App that allows packs of additional images to be provided for inclusion in the message content.
iMessage Extension
Allows interactive content to be integrated into the Messages app. This can range from custom user interfaces to interactive games. iMessage extensions are covered in the chapters entitled An Introduction to Building iOS 17 Message Apps and An iOS 17 Interactive Message App Tutorial.
Intents Extension
When integrating an app with the SiriKit framework, these extensions define the actions to be performed in response to voice commands using Siri. SiriKit integration and intents extensions are covered beginning with the chapter entitled An Introduction to iOS 17 Sprite Kit Programming.
Creating Extensions
By far, the easiest approach to developing extensions is to use the extension templates provided by Xcode. Once the project for a containing app is loaded into Xcode, extensions can be added as new targets by selecting the File -> New -> Targets… menu option. This will display the panel shown in Figure 75-3, listing a template for each of the extension types:
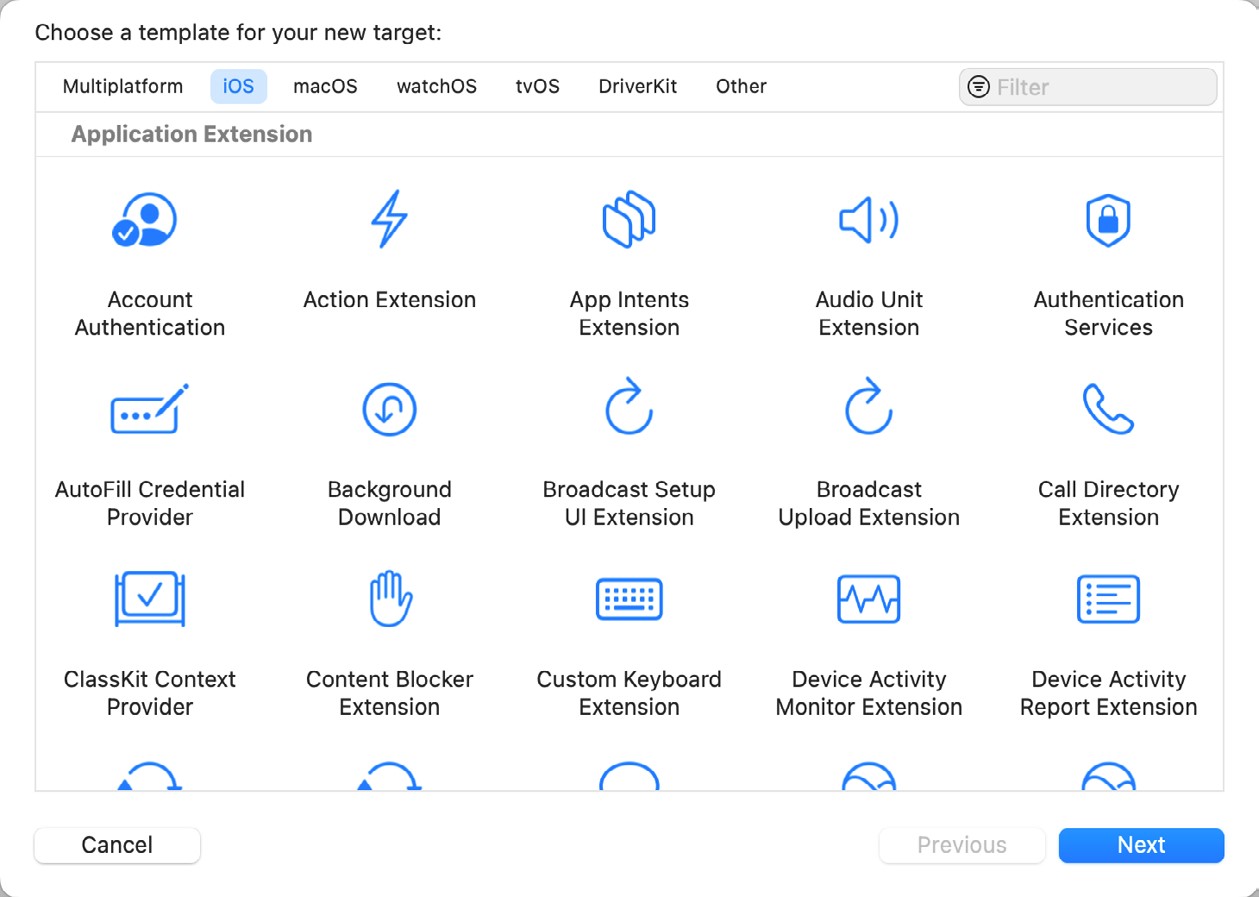
Once an extension template is selected, click on Next to name and create the template. Once the extension has been created from the template, the steps to implement the extension will differ depending on the type of extension selected. The next few chapters will detail the steps in implementing Photo Editing, Action, and Message app extensions.
Summary
Extensions in iOS provide a way for narrowly defined areas of functionality of one app to be made available from within other apps. iOS 17 currently supports a variety of extension types. When developing extensions, it is important to select the most appropriate extension point before beginning development work and to be aware that some app features may not be appropriate candidates for an extension.
Although extensions run as separate independent binaries, they can only be installed as part of an app bundle. The app with which an extension is bundled is called a containing app. An app from which an extension is launched is called a host app.
Having covered the basics of extensions in this chapter, subsequent chapters will focus in detail on the more commonly used extension types.