When a new SwiftUI project is created in Xcode using the Multiplatform App template, Xcode generates a collection of files and folders that form the basis of the project and on which the finished app will eventually be built.
Although it is not necessary to know in detail about the purpose of each of these files when beginning with SwiftUI development, each of them will become useful as you develop more complex applications. This chapter will briefly overview each basic Xcode SwiftUI project structure element.
Creating an Example Project
If you have not already done so, creating a sample project to review may be helpful while working through this chapter. To do so, launch Xcode and, on the welcome screen, select the option to create a new project. On the resulting template selection panel, select the Multiplatform tab followed by the App option before proceeding to the next screen:
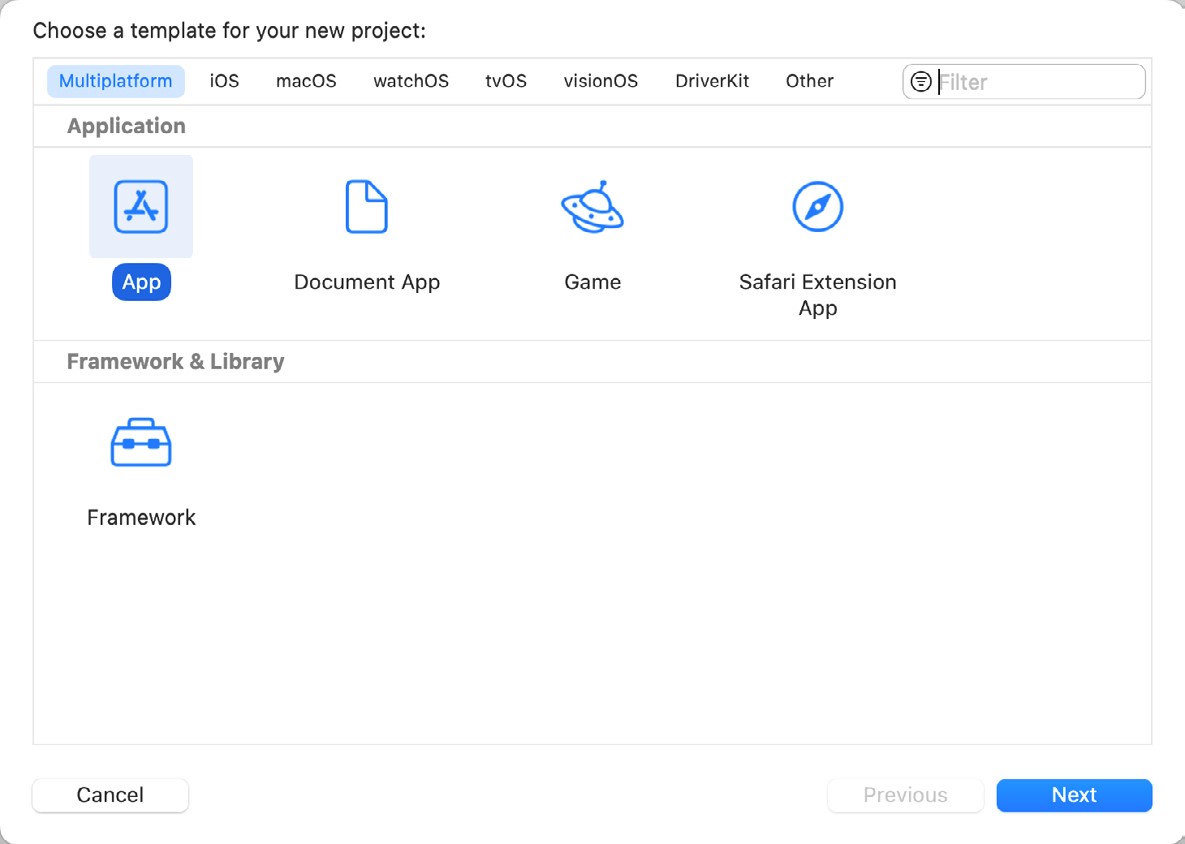
On the project options screen, name the project DemoProject. Click Next to proceed to the final screen, choose a suitable filesystem location for the project, and click the Create button.
The DemoProjectApp.swift File
The DemoProjectApp.swift file contains the declaration for the App object as described in the chapter entitled SwiftUI Architecture and will read as follows:
import SwiftUI
@main
struct DemoProjectApp: App {
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
Code language: Swift (swift)
As implemented, the declaration returns a Scene consisting of a WindowGroup containing the View defined in the ContentView.swift file. Note that the declaration is prefixed with @main. This indicates to SwiftUI that this is the entry point for the app when it is launched on a device.
The ContentView.swift File
This is a SwiftUI View file that, by default, contains the content of the first screen to appear when the app starts. This file and others like it are where most of the work is performed when developing apps in SwiftUI. By default, it contains an Image view and a Text view displaying the words “Hello, world!” arranged within a vertical stack container:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Image(systemName: "globe")
.imageScale(.large)
.foregroundStyle(.tint)
Text("Hello, world!")
}
.padding()
}
}
#Preview {
ContentView()
}
Code language: Swift (swift)
Assets.xcassets
The Assets.xcassets folder contains the asset catalog that stores resources used by the app, such as images, icons, and colors.
DemoProject.entitlements
The entitlements file is used to enable support for specific iOS features within the app. For example, if your app needs access to iCloud storage or the device microphone, or if you plan to integrate voice control via Siri, these entitlements must be enabled within this file.
Preview Content
The preview assets folder contains any assets and data needed when previewing the app during development but not required in the completed app. When you are ready to package your app for submission to the app store, Xcode will remove these assets from the delivery archive so they do not take up unnecessary space.
Summary
When a new SwiftUI project is created in Xcode using the Multiplatform App template, Xcode automatically generates the minimal files required for the app to function. All of these files and folders can be modified to add functionality to the app, both in terms of adding resource assets, performing initialization and de-initialization tasks, and building the user interface and logic of the app.