Now that we have a better idea of what composable functions are and how to create them, it is time to explore composables that provide a slot API. In this chapter, we will explain what a slot API is, what it is used for and how you can include slots in your own composable functions. We will also explore some of the built-in composables that provide slot API support.
Understanding slot APIs
As we already know, composable functions can include calls to one or more other composable functions. This usually means that the content of a composable is predefined in terms of which other composables it calls and, therefore, the content it displays. Consider the following function consisting of a Column and three Text components:
@Composable
fun SlotDemo() {
Column {
Text("Top Text")
Text("Middle Text")
Text("Bottom Text")
}
}
Code language: Kotlin (kotlin)
The function could be modified to pass in parameters that specify the text to be displayed or even the color and font size of that text. Regardless of the changes we make, however, the function is still restricted to displaying a column containing three Text components:
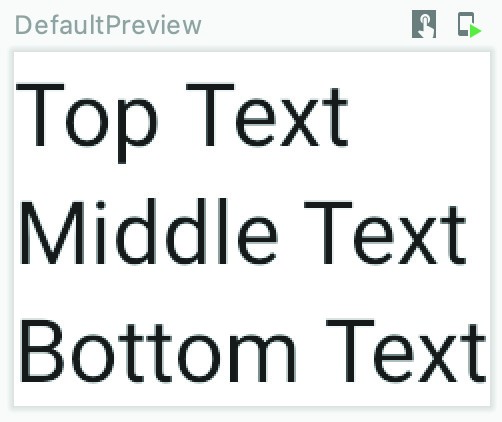
Figure 22-1
Suppose, however, that we need to display three items in a column, but do not know what composable will take up the middle position until just before the composable is called. In its current form, there is no way to display anything but the declared Text component in the middle position. The solution to this problem is to open up the middle composable as a slot into which any other composable may be placed when the function is called. This is referred to as providing a slot API for the composable. API is an abbreviation of Application Programming Interface and, in this context, implies that we are adding a programming interface to our composable that allows the caller to specify the composable to appear within a slot. In fact, a composable function can provide multiple slots to the caller. In the above function, for example, all of the Text components could be declared as slots if required.
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |
Declaring a slot API
It can be helpful to think of a slot API composable as a user interface template in which one or more elements are left blank. These missing pieces are then passed as parameters when the composable is called and included when the user interface is rendered by the Compose runtime system.
The first step in adding slots to a composable is to specify that it accepts a slot as a parameter. This is essentially a case of declaring that a composable accepts other composables as parameters. In the case of our example SlotDemo composable, we would modify the function signature as follows:
@Composable
fun SlotDemo(middleContent: @Composable () -> Unit) {
.
.
Code language: Kotlin (kotlin)
When the SlotDemo composable is called, it will now need to be passed a composable function. Note that the function is declared as returning a Unit object. Unit is a Kotlin type used to indicate that a function does not return any value. Unit can be considered to be the Kotlin equivalent of void in other languages. The parameter has been assigned a label of “middleContent”, though this could be any valid label name that helps to describe the slot and allows us to reference it within the body of the function.
The only remaining change to this composable is to substitute the middleContent component into the Column declaration as follows:
@Composable
fun SlotDemo(middleContent: @Composable () -> Unit) {
Column {
Text("Top Text")
middleContent()
Text("Bottom Text")
}
}
Code language: Kotlin (kotlin)
We have now successfully declared a slot API for our SlotDemo composable.
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |
Calling slot API composables
The next step is to learn how to make use of the slot API configured into our SlotDemo composable. This simply involves passing a composable through as a parameter when making the SlotDemo function call. Suppose, for example, that we need the following composable to appear in the middleContent slot:
@Composable
fun ButtonDemo() {
Button(onClick = { }) {
Text("Click Me")
}
}
Code language: Kotlin (kotlin)
We can now call our SlotDemo composable function as follows:
SlotDemo(middleContent = { ButtonDemo() })
Code language: Kotlin (kotlin)
While this syntax works, it can quickly become cluttered if the composable has more than one slot to be filled. A cleaner syntax reads as follows:
SlotDemo {
ButtonDemo()
}
Code language: Kotlin (kotlin)
Regardless of the syntax used, the design will be rendered as shown below in Figure 22-2:
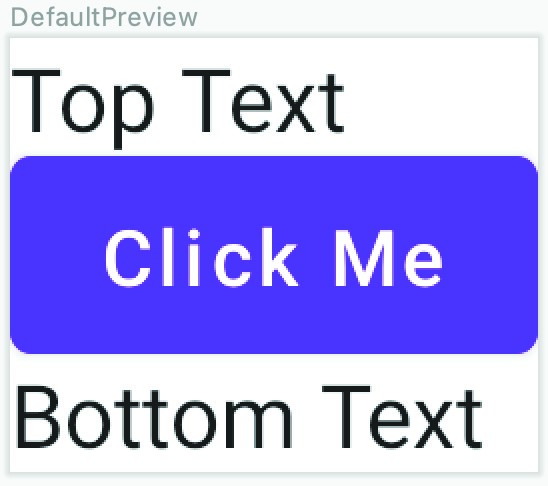
Figure 22-2
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |
A slot API is not, of course, limited to a single slot. The SlotDemo example could be composed entirely of slots as follows:
@Composable
fun SlotDemo(
topContent: @Composable () -> Unit,
middleContent: @Composable () -> Unit,
bottomContent: @Composable () -> Unit) {
Column {
topContent()
middleContent()
bottomContent()
}
}
Code language: Kotlin (kotlin)
With these changes made, the call to SlotDemo could be structured as follows:
SlotDemo(
topContent = { Text("Top Text") },
middleContent = { ButtonDemo() },
bottomContent = { Text("Bottom Text") }
)
Code language: Kotlin (kotlin)
As with the single slot, this can be abbreviated for clarity:
SlotDemo(
{ Text("Top Text") },
{ ButtonDemo() },
{ Text("Bottom Text") }
)
Code language: Kotlin (kotlin)
Summary
In this chapter, we have introduced the concept of slot APIs and demonstrated how they can be added to composable functions. By implementing a slot API, the content of a composable function can be specified dynamically at the point that it is called. This contrasts with the static content of a typical composable where the content is defined at the point the function is written and cannot subsequently be changed. A composable with a slot API is essentially a user interface template containing one or more slots into which other composables can be inserted at runtime.
With the basics of slot APIs covered in this chapter, the next chapter will create a project that puts this theory into practice.
![]() |
You are reading a sample chapter from an old edition of the Jetpack Compose Essentials book. Purchase the fully updated Jetpack Compose 1.7 Essentials edition of this book in eBook or Print format. The full book contains 67 chapters and over 700 pages of in-depth information. Buy the full book now in Print or eBook format. Learn more. |