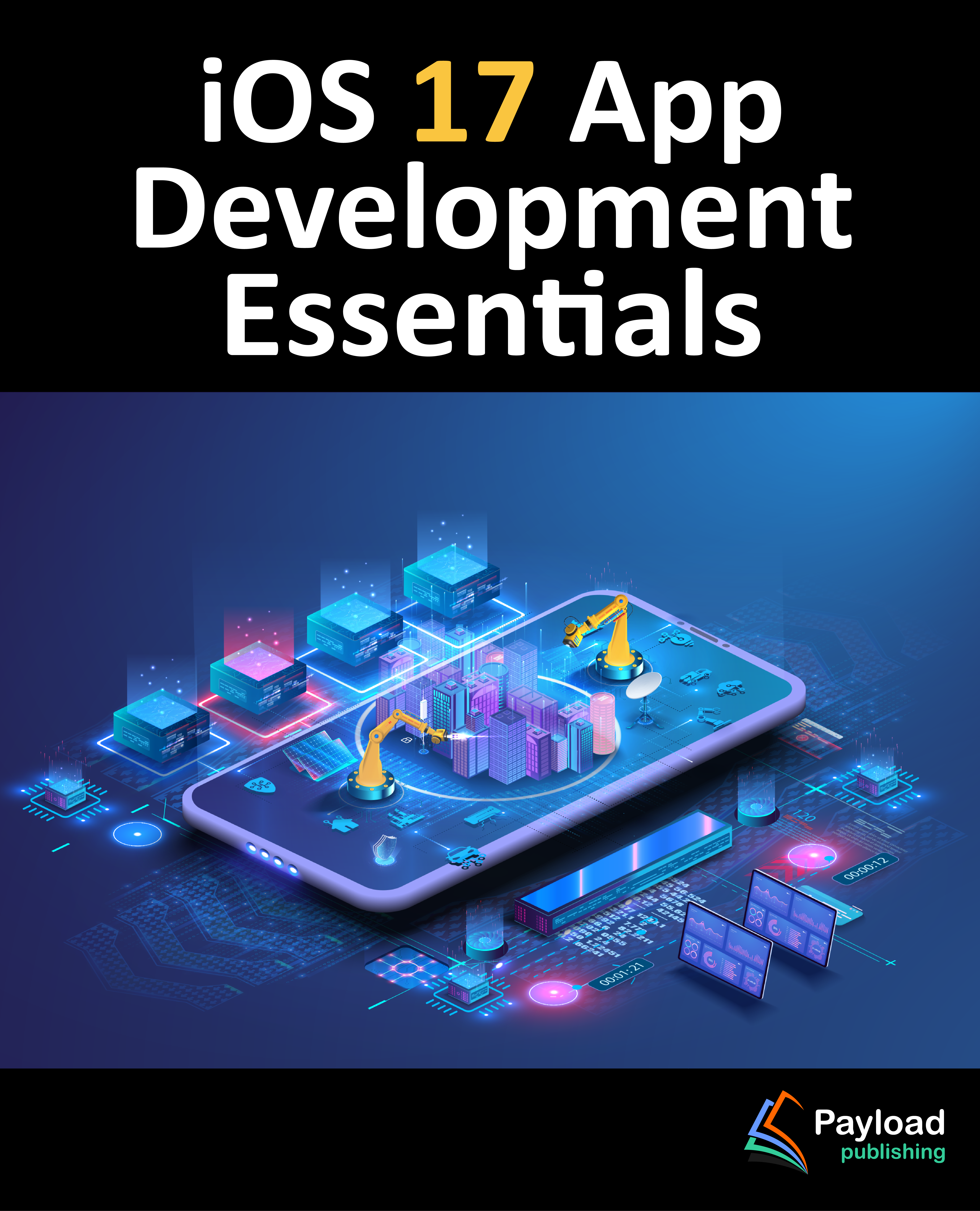
This collection of multiple-choice questions is designed to test your understanding of Swift programming, focusing on data types, constants, and variables. Each question addresses key concepts and fundamentals necessary for effective programming in Swift.