Before moving on from touch handling in general and gesture recognition in particular, the topic of this chapter is handling pinch gestures. While it is possible to create and detect a wide range of gestures using the steps outlined in the previous sections of this chapter, it is, in fact, not possible to detect a pinching gesture (where two fingers are used in a stretching and pinching motion, typically to zoom in and out of a view or image) using the techniques discussed so far.
The simplest method for detecting pinch gestures is to use the Android ScaleGestureDetector class. In general terms, detecting pinch gestures involves the following three steps:
- Declaration of a new class which implements the SimpleOnScaleGestureListener interface, including the required onScale(), onScaleBegin(), and onScaleEnd() callback methods.
- Creation of an instance of the ScaleGestureDetector class, passing through an instance of the class created in step 1 as an argument.
- Implementing the onTouchEvent() callback method on the enclosing activity, which, in turn, calls the onTouchEvent() method of the ScaleGestureDetector class.
In the remainder of this chapter, we will create an example designed to demonstrate the implementation of pinch gesture recognition.
A Pinch Gesture Example Project
Select the New Project option from the welcome screen and, within the resulting new project dialog, choose the Empty Views Activity template before clicking on the Next button.
Enter PinchExample into the Name field and specify com.ebookfrenzy.pinchexample as the package name. Before clicking on the Finish button, change the Minimum API level setting to API 26: Android 8.0 (Oreo) and the Language menu to Java. Convert the project to use view binding by following the steps in 11.8 Migrating a Project to View Binding.
Within the activity_main.xml file, select the default TextView object and use the Attributes tool window to set the ID to myTextView.
Locate and load the MainActivity.java file into the Android Studio editor and modify the file as follows:
package com.ebookfrenzy.pinchexample;
.
.
import android.view.MotionEvent;
import android.view.ScaleGestureDetector;
import android.view.ScaleGestureDetector.SimpleOnScaleGestureListener;
public class MainActivity extends AppCompatActivity {
private ActivityMainBinding binding;
ScaleGestureDetector scaleGestureDetector;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
binding = ActivityMainBinding.inflate(getLayoutInflater());
View view = binding.getRoot();
setContentView(view);
scaleGestureDetector =
new ScaleGestureDetector(this,
new MyOnScaleGestureListener());
}
@Override
public boolean onTouchEvent(MotionEvent event) {
scaleGestureDetector.onTouchEvent(event);
return true;
}
public class MyOnScaleGestureListener extends
SimpleOnScaleGestureListener {
@Override
public boolean onScale(ScaleGestureDetector detector) {
float scaleFactor = detector.getScaleFactor();
if (scaleFactor > 1) {
binding.myTextView.setText("Zooming In");
} else {
binding.myTextView.setText("Zooming Out");
}
return true;
}
@Override
public boolean onScaleBegin(ScaleGestureDetector detector) {
return true;
}
@Override
public void onScaleEnd(ScaleGestureDetector detector) {
}
}
.
.
.
}
Code language: Java (java)
The code declares a new class named MyOnScaleGestureListener, extending the Android
SimpleOnScaleGestureListener class. This interface requires that three methods (onScale(), onScaleBegin(), and onScaleEnd()) be implemented. In this instance, the onScale() method identifies the scale factor and displays a message on the text view indicating the type of pinch gesture detected.
Within the onCreate() method, a new ScaleGestureDetector instance is created, passing through a reference to the enclosing activity and an instance of our new MyOnScaleGestureListener class as arguments. Finally, an onTouchEvent() callback method is implemented for the activity, which calls the corresponding onTouchEvent() method of the ScaleGestureDetector object, passing through the MotionEvent object as an argument.
Compile and run the application on an emulator or physical Android device and perform pinching gestures on the screen, noting that the text view displays either the zoom-in or zoom-out message depending on the pinching motion. Pinching gestures may be simulated within the emulator in stand-alone mode by holding down the Ctrl (or macOS Cmd) key and clicking and dragging the mouse pointer, as shown in Figure 36-3:
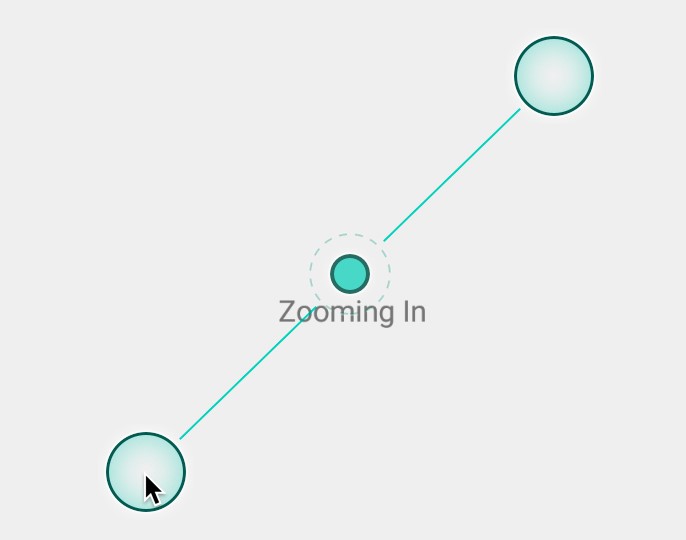